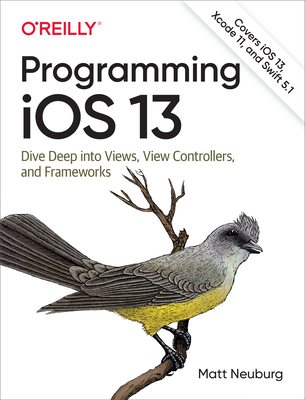
Programming IOS 13: Dive Deep Into Views, View Controllers, and Frameworks
內容描述
If you're grounded in the basics of Swift, Xcode, and the Cocoa framework, this book provides a structured explanation of all essential real-world iOS app components. Through deep exploration and copious code examples, you'll learn how to create views, manipulate view controllers, and add features from iOS frameworks.
Create, arrange, draw, layer, and animate views that respond to touch
Use view controllers to manage multiple screens of interface
Master interface classes for scroll views, table views, text, popovers, split views, web views, and controls
Dive into frameworks for sound, video, maps, and sensors
Access user libraries: music, photos, contacts, and calendar
Explore additional topics, including files, networking, and threads
目錄大綱
How to Contact Us
I. Views
- Views
Window and Root View
How an App Launches
App Without a Storyboard
Referring to the Window
Experimenting with Views
Subview and Superview
Color
Visibility and Opacity
Frame
Bounds and Center
Transform
Transform3D
Window Coordinates and Screen Coordinates
Trait Collections
Interface Style
Size Classes
Overriding Trait Collections
Layout
Autoresizing
Autolayout and Constraints
Implicit Autoresizing Constraints
Creating Constraints in Code
Constraints as Objects
Margins and Guides
Intrinsic Content Size
Self-Sizing Views
Stack Views
Internationalization
Mistakes with Constraints
Configuring Layout in the Nib
Autoresizing in the Nib
Creating a Constraint
Viewing and Editing Constraints
Problems with Nib Constraints
Varying the Screen Size
Conditional Interface Design
Xcode View Features
View Debugger
Previewing Your Interface
Designable Views and Inspectable Properties
Layout Events - Drawing
Images and Image Views
Image Files
Image Views
Resizable Images
Transparency Masks
Reversible Images
Graphics Contexts
Drawing on Demand
Drawing a UIImage
UIImage Drawing
CGImage Drawing
Snapshots
CIFilter and CIImage
Blur and Vibrancy Views
Drawing a UIView
Graphics Context Commands
Graphics Context Settings
Paths and Shapes
Clipping
Gradients
Colors and Patterns
Graphics Context Transforms
Shadows
Erasing
Points and Pixels
Content Mode - Layers
View and Layer
Layers and Sublayers
Manipulating the Layer Hierarchy
Positioning a Sublayer
CAScrollLayer
Layer and Delegate
Layout of Layers
Drawing in a Layer
Drawing-Related Layer Properties
Content Resizing and Positioning
Layers that Draw Themselves
Transforms
Affine Transforms
3D Transforms
Depth
Further Layer Features
Shadows
Borders and Rounded Corners
Masks
Layer Efficiency
Layers and Key–Value Coding - Animation
Drawing, Animation, and Threading
Image View and Image Animation
View Animation
A Brief History of View Animation
Property Animator Basics
View Animation Basics
View Animation Configuration
Timing Curves
Canceling a View Animation
Frozen View Animation
Custom Animatable View Properties
Keyframe View Animation
Transitions
Implicit Layer Animation
Animatable Layer Properties
Animation Transactions
Media Timing Functions
Core Animation
CABasicAnimation and Its Inheritance
Using a CABasicAnimation
Springing Animation
Keyframe Animation
Making a Property Animatable
Grouped Animations
Freezing an Animation
Transitions
Animations List
Actions
What an Action Is
Action Search
Hooking Into the Action Search
Making a Custom Property Implicitly Animatable
Nonproperty Actions
Emitter Layers
CIFilter Transitions
UIKit Dynamics
The Dynamics Stack
Custom Behaviors
Animator and Behaviors
Motion Effects
Animation and Layout - Touches
Touch Events and Views
Receiving Touches
Restricting Touches
Interpreting Touches
Gesture Recognizers
Gesture Recognizer Classes
Gesture Recognizer Conflicts
Gesture Recognizer Delegate
Subclassing Gesture Recognizers
Gesture Recognizers in the Nib
3D Touch Press Gesture
Touch Delivery
Hit-Testing
Performing Hit-Testing
Hit-Test Munging
Hit-Testing for Layers
Hit-Testing for Drawings
Hit-Testing During Animation
Initial Touch Event Delivery
Gesture Recognizer and View
Touch Exclusion Logic
Gesture Recognition Logic
II. Interface - View Controllers
View Controller Responsibilities
View Controller Hierarchy
Automatic Child View Placement
Manual Child View Placement
Presented View Placement
Ensuring a Coherent Hierarchy
View Controller Creation
How a View Controller Obtains Its View
Manual View
Generic Automatic View
View in a Separate Nib
Summary
How Storyboards Work
How a Storyboard View Controller Nib is Loaded
How a Storyboard View Nib is Loaded
View Resizing
View Size in the Nib Editor
Bars and Underlapping
Resizing and Layout Events
Rotation
Uses of Rotation
Permitting Compensatory Rotation
Initial Orientation
Detecting Rotation
View Controller Manual Layout
Initial Manual Layout
Manual Layout During Rotation
Presented View Controller
Presentation and Dismissal
Configuring a Presentation
Communication with a Presented View Controller
Adaptive Presentation
Presentation, Rotation, and the Status Bar
Tab Bar Controller
Tab Bar Items
Configuring a Tab Bar Controller
Navigation Controller
Bar Button Items
Navigation Items and Toolbar Items
Configuring a Navigation Controller
Custom Transition
Noninteractive Custom Transition Animation
Interactive Custom Transition Animation
Custom Presented View Controller Transition
Transition Coordinator
Page View Controller
Preparing a Page View Controller
Page View Controller Navigation
Other Page View Controller Configurations
Container View Controllers
Adding and Removing Children
Status Bar, Traits, and Resizing
Previews and Context Menus
Storyboards
Triggered Segues
Container Views and Embed Segues
Storyboard References
Unwind Segues
View Controller Lifetime Events
Order of Events
Appear and Disappear Events
Event Forwarding to a Child View Controller
View Controller Memory Management
Lazy Loading
NSCache, NSPurgeableData, and Memory-Mapping
Background Memory Usage - Scroll Views
Content Size
Creating a Scroll View in Code
Manual Content Size
Automatic Content Size with Autolayout
Scroll View Layout Guides
Using a Content View
Scroll View in a Nib
Content Inset
Scrolling
Scrolling in Code
Paging
Tiling
Zooming
Zooming Programmatically
Zooming with Detail
Scroll View Delegate
Scroll View Touches
Floating Scroll View Subviews
Scroll View Performance - Table Views and Collection Views
Table View Controller
Table View Cells
Built-In Cell Styles
Registering a Cell Class
Custom Cells
Table View Data
The Three Big Questions
Reusing Cells
Table View Sections
Section Headers and Footers
Table View Section Example
Section Index
Variable Row Heights
Manual Row Height Measurement
Measurement and Layout with Constraints
Estimated Height
Automatic Row Height
Table View Selection
Managing Cell Selection
Responding to Cell Selection
Navigation from a Table View
Table View Scrolling and Layout
Refreshing a Table View
Cell Choice and Static Tables
Direct Access to Cells
Refresh Control
Editing a Table View
Toggling a Table View’s Edit Mode
Edit Mode and Selection
Changing a Table View’s Structure
Deleting a Cell
Deleting Multiple Cells
Table View Diffable Data Source
Populating a Diffable Data Source
Subclassing a Diffable Data Source
Changing a Diffable Data Source
Pros and Cons of the Diffable Data Source
More Table View Editing
Rearranging Cells
Editable Content in Cells
Expandable Cell
Table View Swipe Action Buttons
Table View Menus
Table View Searching
Configuring a Search Controller
Using a Search Controller
Collection Views
Collection View Classes
Flow Layout
Compositional Layout
Size, Count, Spacing, and Insets
Supplementary Items
Multiple Section Layouts
Other Compositional Layout Features
Collection View Diffable Data Source
Basic Cell Manipulation
Selecting Cells
Deleting Cells
Menu Handling
Rearranging Cells
Custom Collection View Layouts
Tweaking a Layout
Collection View Layout Subclass
Decoration Views
Switching Layouts
Collection Views and UIKit Dynamics - iPad Interface
Popovers
Arrow Source and Direction
Popover Size
Popover Appearance
Passthrough Views
Popover Presentation, Dismissal, and Delegate
Adaptive Popovers
Popover Segues
Popover Presenting a View Controller
Split Views
Expanded Split View Controller (iPad)
Collapsed Split View Controller (iPhone)
Expanding Split View Controller (Big iPhone)
Customizing a Split View Controller
Split View Controller in a Storyboard
Setting the Collapsed State
View Controller Message Percolation
iPad Multitasking
Drag and Drop
Drag and Drop Architecture
Basic Drag and Drop
Item Providers
Slow Data Delivery
Additional Delegate Methods
Table Views and Collection Views
Spring Loading
iPhone and Local Drag and Drop
Multiple Windows
The Window Architecture
Scene Creation
Window Creation and Closing
State Saving and Restoration
Further Multiple Window Considerations - Text
Fonts and Font Descriptors
Fonts
Symbol Images and Text
Font Descriptors
Choosing a Font
Adding Fonts
Attributed Strings
Attributed String Attributes
Making an Attributed String
Modifying and Querying an Attributed String
Custom Attributes
Drawing and Measuring an Attributed String
Labels
Number of Lines
Wrapping and Truncation
Fitting Label and Text
Customized Label Drawing
Text Fields
Summoning and Dismissing the Keyboard
Keyboard Covers Text Field
Text Field Delegate and Control Event Messages
Text Field Menu
Drag and Drop
Keyboard and Input Configuration
Text Views
Links, Text Attachments, and Data
Self-Sizing Text View
Text View and Keyboard
Text Kit
Text View and Text Kit
Text Container
Alternative Text Kit Stack Architectures
Layout Manager
Text Kit Without a Text View - Web Views
WKWebView
Web View Content
Tracking Changes in a Web View
Web View Navigation
Communicating with a Web Page
Custom Schemes
Web View Previews and Context Menus
Safari View Controller
Developing Web View Content - Controls and Other Views
UIActivityIndicatorView
UIProgressView
Progress View Alternatives
The Progress Class
UIPickerView
UISearchBar
UIControl
UISwitch
UIStepper
UIPageControl
UIDatePicker
UISlider
UISegmentedControl
UIButton
Custom Controls
Bars
Bar Position
Bar Metrics
Bar and Item Appearance
Bar Background and Shadow
Bar Button Items
Navigation Bar
Toolbar
Tab Bar
Tint Color
Appearance Proxy - Modal Dialogs
Alerts and Action Sheets
Alerts
Action Sheets
Alert Alternatives
Quick Actions
Local Notifications
Authorization for Local Notifications
Notification Categories
Scheduling a Local Notification
Hearing About a Local Notification
Grouped Notifications
Managing Notifications
Notification Content Extensions
Today Extensions
Activity Views
Presenting an Activity View
Custom Activities
Action Extensions
Share Extensions
III. Some Frameworks - Audio
System Sounds
Audio Session
Category
Activation and Deactivation
Ducking
Interruptions
Secondary Audio
Routing Changes
Audio Player
Remote Control of Your Sound
Playing Sound in the Background
AVAudioRecorder
AVAudioEngine
MIDI Playback
Text to Speech
Speech to Text
Further Topics in Sound - Video
AVPlayerViewController
Other AVPlayerViewController Properties
Picture-in-Picture
Introducing AV Foundation
Some AV Foundation Classes
Things Take Time
Time Is Measured Oddly
Constructing Media
AVPlayerLayer
Further Exploration of AV Foundation
UIVideoEditorController - Music Library
Music Library Authorization
Exploring the Music Library
Querying the Music Library
Persistence and Change in the Music Library
Music Player
Setting the Queue
Modifying the Queue
Player State
MPVolumeView
Playing Songs with AV Foundation
Media Picker - Photo Library and Camera
Browsing with UIImagePickerController
Image Picker Controller Presentation
Image Picker Controller Delegate
Dealing with Image Picker Controller Results
Photos Framework
Querying the Photo Library
Modifying the Library
Being Notified of Changes
Fetching Images
Editing Images
Photo Editing Extension
Using the Camera
Capture with UIImagePickerController
Capture with AV Foundation - Contacts
Contact Classes
Fetching Contact Information
Fetching a Contact
Repopulating a Contact
Labeled Values
Contact Formatters
Saving Contact Information
Contact Sorting, Groups, and Containers
Contacts Interface
CNContactPickerViewController
CNContactViewController - Calendar
Calendar Database Contents
Calendars
Calendar Items
Calendar Database Changes
Creating Calendars, Events, and Reminders
Events
Alarms
Recurrence
Reminders
Proximity Alarms
Fetching Events and Reminders
Calendar Interface
EKEventViewController
EKEventEditViewController
EKCalendarChooser - Maps
Map Views
Displaying a Region
Scrolling and Zooming
Other Map View Customizations
Map Images
Annotations
Customizing an MKMarkerAnnotationView
Changing the Annotation View Class
Custom Annotation View Class
Custom Annotation Class
Annotation View Hiding and Clustering
Other Annotation Features
Overlays
Custom Overlay Class
Custom Overlay Renderer
Other Overlay Features
Map Kit and Current Location
Communicating with the Maps App
Geocoding, Searching, and Directions
Geocoding
Searching
Directions - Sensors
Core Location
Location Manager and Delegate
Location Services Authorization
Location Tracking
Where Am I?
Continuous Background Location
Location Monitoring
Heading
Acceleration, Attitude, and Activity
Shake Events
Using Core Motion
Raw Acceleration
Gyroscope
Other Core Motion Data
IV. Final Topics - Persistent Storage
The Sandbox
Standard Directories
Inspecting the Sandbox
Basic File Operations
Saving and Reading Files
File Coordinators
File Wrappers
User Defaults
Simple Sharing and Previewing of Files
File Sharing
Document Types and Receiving a Document
Handing Over a Document
Previewing a Document
Quick Look Previews
Document Architecture
A Basic Document Example
iCloud
Document Browser
Custom Thumbnails
Custom Previews
Document Picker
XML
JSON
Coding Keys
Custom Decoding
SQLite
Core Data
PDFs
Image Files - Basic Networking
HTTP Requests
Obtaining a Session
Session Configuration
Session Tasks
Session Delegate
HTTP Request with Task Completion Function
HTTP Request with Session Delegate
One Session, One Delegate
Delegate Memory Management
Session and Delegate Encapsulation
Downloading Table View Data
Background Session
On-Demand Resources
In-App Purchases - Threads
Main Thread
Background Threads
Why Threading Is Hard
Blocking the Main Thread
Manual Threading
Operation
Grand Central Dispatch
Commonly Used GCD Methods
Synchronous Execution
Dispatch Groups
One-Time Execution
Concurrent Queues
Checking the Queue
App Backgrounding
Background Processing - Undo
Target–Action Undo
Undo Grouping
Functional Undo
Undo Interface
Shake-To-Edit
Built-In Gestures
Undo Menu
A. Lifetime Events
Application States
Delegate Events
Lifetime Scenarios
Major State Changes
Paused Inactivity
Transient Inactivity on the iPad
Multiple Windows
Scene Death in the App Switcher
Lifetime Event Timing
B. Some Useful Utility Functions
Core Graphics Initializers
Center of a CGRect
Adjust a CGSize
Delayed Performance
Dictionary of Views
Constraint Issues
Named Views
Subviews of Given Class
Configure a Value Class at the Point of Use
Downsize a UIImage
C. How Asynchronous Works
Index
作者介紹
Matt Neuburg has a PhD in Classics and has taught at many universities and colleges. He has been programming computers since 1968. He has written applications for Mac OS X and iOS, is a former editor of MacTech Magazine, and is a long-standing contributing editor for TidBITS. His previous O'Reilly books are Frontier: The Definitive Guide, REALbasic: The Definitive Guide, and AppleScript: The Definitive Guide. He makes a living writing books, articles, and software documentation, as well as by programming, consulting, and training.